Encountering a “SyntaxError: Cannot use import statement outside a module” can be confusing, especially if you’re just starting with JavaScript or building a Node.js or front-end project using modern module syntax. This error typically happens when the JavaScript engine tries to interpret an ES6 import
statement in a script context that hasn’t been properly instructed to treat the file as a module. Fortunately, fixing this error is straightforward once you understand a few key concepts.
Understanding the Error
The error message points to a fundamental mismatch: you are using an ES6 module syntax (import
) in a script that the runtime does not recognize as a module. The JavaScript runtime divides files into either traditional scripts or modules. By default, browsers and Node.js treat files as scripts unless told otherwise.
For example, you might see an error like this:
SyntaxError: Cannot use import statement outside a module
This means one of two things:
- The JavaScript file is not being loaded as a module.
- The environment does not support ES6 modules the way you’re using them.
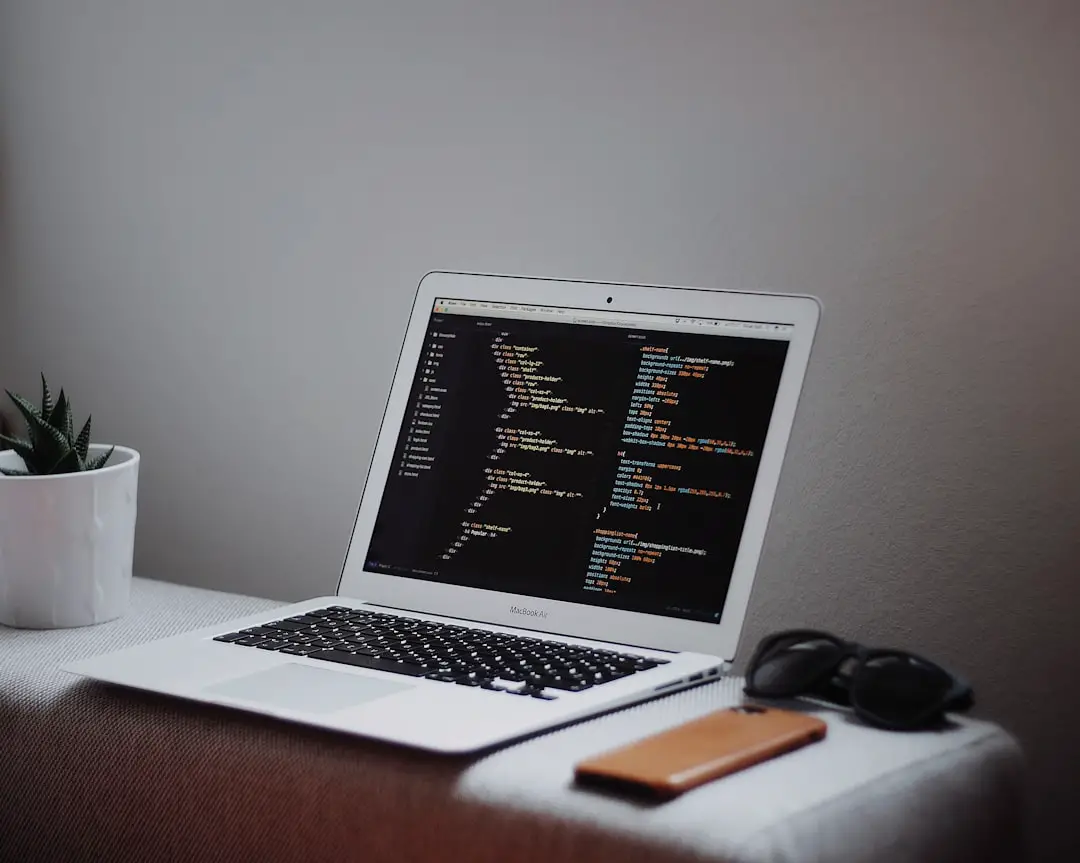
Fixing the Error in Browser-Based Projects
If you’re working on a browser project and getting this error, the most common solution is to update your <script>
tag:
<script type="module" src="main.js"></script>
This tells the browser that it should treat main.js
as a module file, allowing you to safely use import
and export
statements.
Other Common Browser Fixes:
- Check your script path: Make sure the
src
attribute is pointing to the correct path. Incorrect paths will also produce confusing errors. - Serve files properly: If you’re opening your HTML file directly in a browser using the
file://
protocol, modules might not work as expected. Use a local server instead (likeLive Server
in VS Code).
Fixing the Error in Node.js Projects
Node.js does not treat JavaScript files as ES modules by default. There are a couple of ways to remedy this:
Option 1: Rename the file to .mjs
You can simply rename your file extension from .js
to .mjs
. Node.js will then treat the file as an ES Module, and allow the use of import
and export
.
Option 2: Configure package.json
Alternatively, you can specify that your project uses modules by adding the following line to your package.json
.
{
"type": "module"
}
Once set, files with a .js
extension will be treated as ES modules automatically.
When to Use require()
Instead
If you’re not ready to switch fully to ES modules, you can always use the CommonJS syntax:
const example = require('./example');
This is especially relevant if you are using older Node.js versions or third-party libraries that haven’t updated to support ES modules.
Using Bundlers like Webpack or Rollup
If you’re building more complex applications, you’re likely using bundlers like Webpack, Rollup, or Vite. These tools allow you to write ES module syntax while seamlessly converting your code to work across environments.
Tips for Bundler Users:
- Ensure your bundler is configured to recognize and transpile
import
syntax. - Install a transpiler like Babel for older browser support.
- Double-check your entry file and plugin configurations.
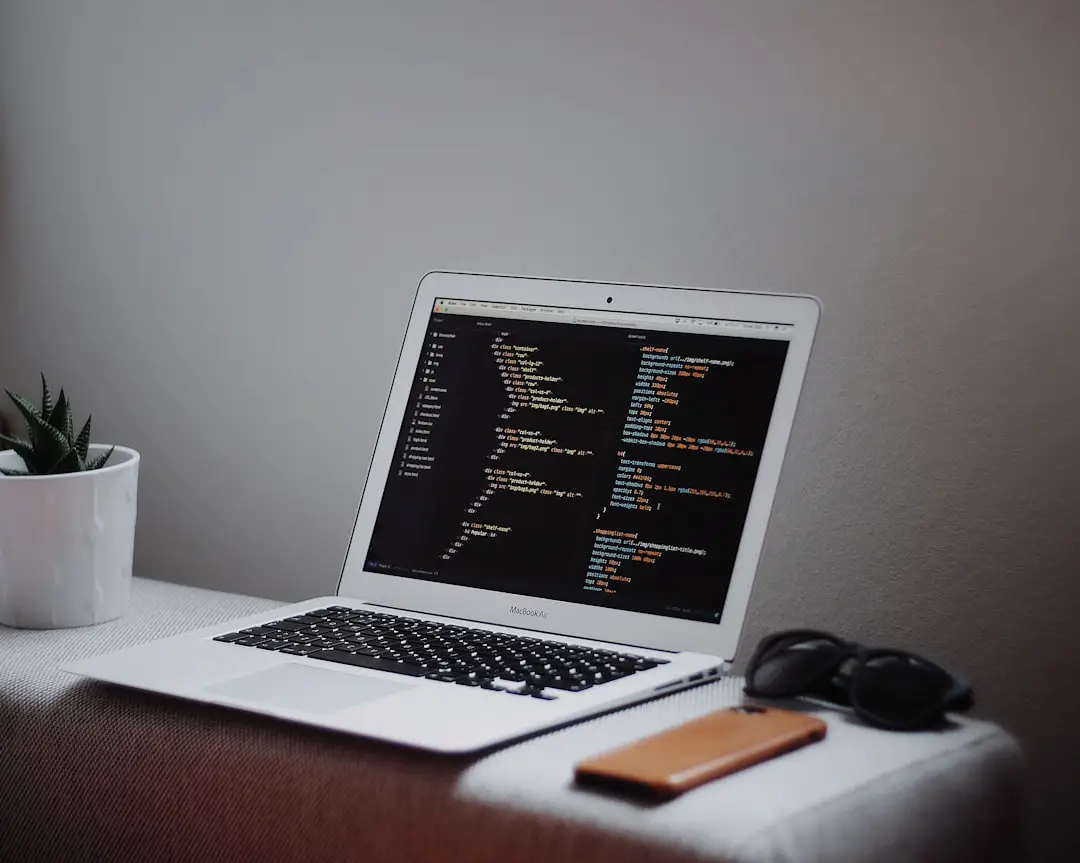
Summary
Fixing the “Cannot use import statement outside a module” error boils down to ensuring that your runtime or environment understands that you’re using module syntax. Whether you’re in the browser, Node.js, or using a bundler, the fix typically involves:
- Marking scripts correctly (with
type="module"
in HTML ortype
inpackage.json
). - Adjusting file extensions to
.mjs
when necessary. - Using a build tool that supports modules properly.
The ES6 module system offers a powerful way to organize and reuse code across JavaScript applications. Once you’ve resolved this error, you’ll find that modules can greatly improve the maintainability and scalability of your codebase.